Many times in programming we have to check to see if a condition is met and then display a result of some sort. This is where the if else statements come in handy with JavaScript. For instance lets say that you wanted to display something on a certain date. Maybe on New Years Day you want to display something like “Happy New Year”. Let’s look at how this can be accomplished with the if else statements in JavaScript.
Understanding String and Integers
There is a big difference in JavaScript when it comes to stings and integers. If I want to program something mathematical I will use integers. I do this just by entering numbers without quotes. Take a look at this example.
var a = 1; var b = 2; var c = a+b;
The output of the above code would be 3. Now lets take a look if I put the numbers in a “string”.
var a = "1"; var b = "2"; var c = a+b;
The output of this code would be 12 because string cannot be added. In fact “strings” cannot do any math at all.
If Else Statements in JavaScript
Ok now lets talk about conditions. If a condition is met then we want to do something or else we want to do something different. Let’s set up the script.
<p id="foo"></p> <script> var x = document.getElementById('foo'); var a = 1; var b = 2; var c = a + b; if(c > 1){ x.innerHTML='value c is less than 1'; }else{ x.innerHTML='value c is more than 1'; } </script>
Explanation
First we set the id of foo in a p tag. Next like we learned in the last lesson we set out document.getELementById(‘foo’) in a variable called “x”. Then we set the number 1 or “integer” of 1 {not a string} in a variable called a. We did the same thing with the variable called b, we put the number 2 in there. In variable c we totaled a + b together.
In our if statement we checked to see if the total {variable c} was greater than the number 1. We do that with this if(c > 1) . Now that we made a “condition” we have to display something. We do that with our “curly brackets” {} . Inside the curly brackets notice we are getting our variable of “x” with contains our document.getElementById(‘foo’). So we just have to write x.innerHTML=’value c is less than 1′; . We could stop there if we want. However we chose to put in a “else” statement to cover if it is greater than 1. We use the else{} capability in JavaScript. We said else{x.innerHTML=’value c is more than 1′;.
Here is the breakdown.
This is the way the if syntax is always written.
if(condition){result}
Addition of Else
The adding of else is always optional but it always comes after an if statement.
if(condition){result}else{other result}
Other Conditions To Check For In “If” Statement
Lets develop this a little further. Here is a list of conditions that you can use.
- < less than.
- > greater than.
- == equal to.
- != not equal to.
More on == equal to
Why do we have to use 2 equal signs? Because if you use 1 equal sign that means you are setting a variable. When you are using 2 equal signs that means you are checking to see if something equals something. You can also use 3 equal signs to be more exact when you are trying to find out if something equals something. Here are examples.
- = sets a variable.
- == check to see if something equals something.
- In this case you could compare a string to an integer and if they match it would come out true.
- === checks to see if something equals something exactly (strict).
- In this case if you compared a string to an integer it would come out false
- != Means that something is NOT EQUAL to something.
- !== Is a strict NOT EQUAL to something.
Displaying Something On A Certain Date With If Else Statement in JavaScript
There are several things that JavaScript comes packaged with. One of those things are the date. You can get the date of a persons browser with a “new Date()” function in JavaScript. Here is an example.
<p id="foo"><p> <script> var x = document.getElementById('foo'); var y = new Date().toLocaleDateString(); x.innerHTML=y; </script>
You will notice this will output the current date on your browser. Pretty cool huh? Well lets say you wanted to display a message on New Years Day. It will say Happy New Year.
Code Example
<p id="foo"><p> <script> var x = document.getElementById('foo'); var y = new Date().toLocaleDateString(); var z = "1/1/2018"; if(y==z){ x.innerHTML='Happy New Year!'; } </script>
Now lets say you want to display something else the rest of the year. In the following code if it is new years day we will display “Happy New Year”. The rest of the days of the year we will display “Have A Nice Day”.
<p id="foo"><p> <script> var x = document.getElementById('foo'); var y = new Date().toLocaleDateString(); var z = "1/1/2018"; if(y==z){ x.innerHTML='Happy New Year!'; }else{ x.innerHTML='Have A Nice day!'; } </script>
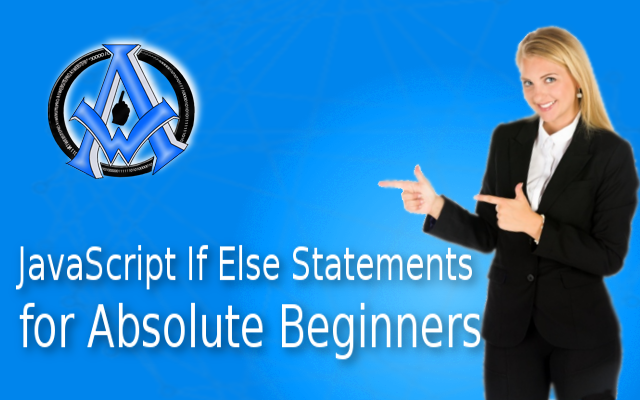

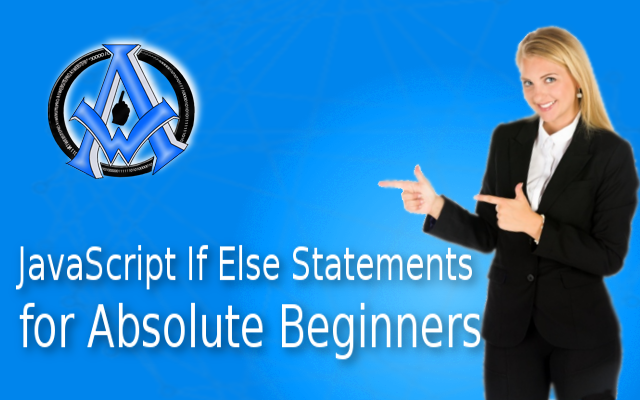
A1WEBSITEPRO Social Media Pages
Here are my social media pages, lets hook up!