Lets discuss DOM events in HTML with JavaScript. In the last lesson the event we were calling upon was the “onclick” event. We used a button to accomplish what we wanted. Today were are going to discuss the following events.
- onclick
- onload
- onchange
- onmouseover
- onmouseout
- onmousedown
- onmouseup
- onfocus
Setting Variables in JavaScript
In the last lesson we were writing document.getElementById(‘foo’).style.color=”red”; over and over again. There is a shorter way to do this. I want you to get use to setting variables in JavaScript because it is going to help you in the future. Instead of writing this syntax over and over again, you can write it one time and store it in a variable. Let me show you.
var x = document.getElementById('foo');
This is all you have to do to set your variable. Of course you would have it in a script tag and possibly a function like we discussed. Now anytime you want to do something with the id of “foo” you can just use an “x”. This is because “x” is holding the entire string of document.getElementById(‘foo’);. So let me write it out for you both ways so you can see the difference.
NOTE: Do not forget your script tags when using the codes below.
The Old Way of Writing JavaScript
document.getElementById('foo').style.color='red'; document.getElementById('foo').style.display='block'; document.getElementById('foo').innerHTML='We change';
Compare to the New Way of Writing JavaScript
var x = document.getElementById('foo'); x.style.color='red'; x.style.display='block'; x.innerHTML='We change';
The Difference in Writing Styles
The difference in these writing styles will give you the advantage when you are writing a lot of different commands to manipulate the DOM.
Calling Onclick Events
To call an onclick event we simply use this syntax inside a tag or element. onclick=””. We have used it in a button before but did you know that we can use it in any element? Check out this code.
<div id="foo" onclick="change()">Hey there</div> function change(){ var x = document.getElementById('foo'); x.style.color='red'; x.innerHTML='We change'; }
Calling Onload Events
The onload event can only happen in the body element. This one is unique this way. You cannot put it in a div or any other element not even the html tag. So if we wanted to load something with the DOM loaded we can use onload in the body tag.
<body onload="change()"> <div id="foo">JavaScript Absolute Beginner Web Development</div> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
Calling Onchange Events
Lets say that you had some forms with input type of text. If you wanted everything to be capitalized and turned red you would have a function like this. This will change after the user enters text and then clicks somewhere else on the page. It will also change if they push the “tab” key on their keyboard.
<input type="text" id="foo" onchange="change()"> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
Calling Onmouseover Events
This event happens when someone put their mouse over an element. The element will have an onmouseover event in it. Here is an example.
<div id="foo" onmouseover="change()">This is a mouse over event.</div> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
Calling Onmouseout Events
You will use this event when you want an event called when a user mouses out of an element on a webpage. Here is an example.
<div id="foo" onmouseout="change()">This is a mouse out event.</div> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
Calling Onmousedown Events
This event will happen when someone pushes their mouse button down. here is an example.
<div id="foo" onmousedown="change()">This is a mouse DOWN event.</div> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
Calling Onmouseup Events
You will notice that this even only takes place when the user releases their mouse button. here is an example of onmouseup event.
<div id="foo" onmouseup="change()">This is a mouse UP event.</div> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
Calling Focus Events
This event is used a lot with forms. When a use clicks or “focuses” on something we can make something happen. Here is an example useing the focus event.
<h1>JavaScript DOM HTML Events for Absolute Beginners</h1> <input type="text" id="foo" onfocus="change()" /> function change(){ var x = document.getElementById('foo'); x.style.color="red"; x.style.backgroundColor="pink"; }
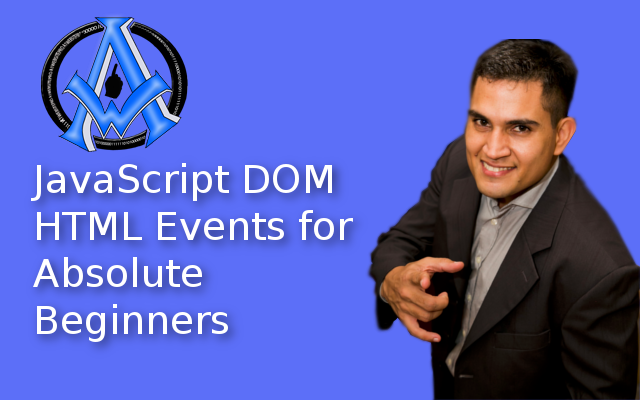

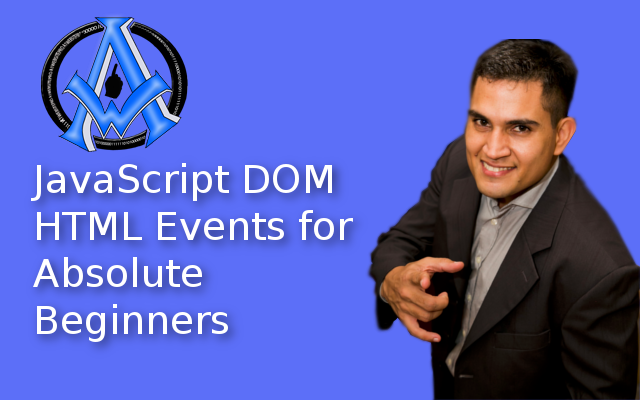
A1WEBSITEPRO Social Media Pages
Here are my social media pages, lets hook up!