When we are programming with PHP remember that it is more artistic like poetry. Everyone will have a different style and you will as well. Many new programmers will not appreciate styles of other programmers because of inexperience. In programming however, housekeeping is very important. New programmers tend to create a lot of messy code. I thought it was important to address this now since we are very early in the programming lessons.
Clean Code in PHP Programming
Here are a couple examples of good and bad code. In this first example you can see that everything is neat and orderly. You can see all the code on the page and the important parts are on different lines so you can make out what the code is doing. Notice that everything takes on a similar structure for easy reading in clean code.
If you compare the 2 code examples below you will start getting the picture. Even though these 2 pieces of code are do the exact same thing one is easy to read while the other is very messy and hard to follow.
So keep your code neat and easy to follow. It will not be exactly as the next guys neat and clean code but it should be understandable and easy to decipher by another programmer.
Example Of Good Clean PHP Code
Example of Messy PHP Code
Making Sense Of PHP Code With Comments
One feature in PHP that you want to make good use of is comments. This is especially true when you are working with a team of programmers or creating an open source application. Many experienced programmers still use comments to keep an efficient workflow. Comments will cancel the operation of PHP code so you must make sure that you put comments on a seperate line.
There are 2 different ways to make comments in PHP. The first one is that you use 2 forward slashes “//”. It is only good for one line of code. It would look something like this.
//This is a comment echo 'Hi There';
The next type of comment is a block comment. You can use this on several lines. It starts with an slash then an asterisk “/*” then ends with an asterisk then a slash. It looks like this.
/*This is a block of code that I am using to comment in PHP. It is very useful when I am programming and need to come back on check something. It is also useful when I am working on projects with a team so they can see what I was doing. */
Cleaning Up Our PHP Code In Our Project
So lets put this into practice in our lessons, shall we? If you are following along open up your project. If you need to download the files for the current lesson download them here. Let’s open up the doo.php page. Currently it looks like this. Let’s take the highlighted portion and create a PHP function with it.
Open up your functions.php file and create this function.
/content for the doo.php page function doopage(){ echo '<h1>Welcome to the doo page<</h1>'; echo '<p>This is the doo page I hope you like it. Love me doo!</p>'; }
Your functions.php file should look like this at this point.
Let’s go back to the doo.php page and insert our function where the content was. The doo.php page should look something like this.
When we load the doo page in the browser we will see that it looks exactly the same as it did.
Let’s do the same thing with our foo.php page. We will have 3 functions by the time we are finished with this page. Take the top part of the page and create a function in the functions.php page like we did for the doo page.
Now let’s call it into the foo.php page right after the header function.
foopage();
Cleaning Up The CSS
When we look at our website we are seeing that it looks a little messy even though the code does not look that bad. In our 18th lesson for the beginner course I taught you how to use css external libraries. We will use bootstrap to clean up our site.
Bring In Bootstrap Library With PHP in Header
Bring up your header.php file. In that file you can see that we are using a function called headtag(). So then bring up the functions.php file and look for the line of code. When you find it change it to this.
function headtag(){ return '<html> <head> <title>My First Programming Website</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <link href="https://fonts.googleapis.com/css?family=Roboto" rel="stylesheet"> <link rel="stylesheet" href="style.css" /> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> </head>'; }
It is important that you always call CSS files before JavaScript files. So when calling in external files like this make sure that the CSS files are on top of the JavaScript files.
Navigate to there header.php and make it look like this. Notice we are calling <div class=”container”> right after the body tag. Bootstrap CSS looks for this tag for formatting purposes.
<?php include_once('functions.php'); echo headtag(); ?> <body><div class="container"> <div class="main" id="top"> <div class="header"> <img src="logo200.png" id="logo" /> </div>
As you know we have to close out that div tag. The best place to close it out would be in the footer.php file. Open your footer.php file and make it look like this.
</div> </body> </html>[
You can see the bootstrap working at you preview it in the browser. Let take care of the menu next. Go to your functions.php and create a function called menu(); Here is what it looks like.
function menu(){ echo '<div class="container"> <nav class="navbar navbar-default"> <div class="container-fluid"> <div class="navbar-header"> <button type="button" class="navbar-toggle" data-toggle="collapse" data-target="#myNavbar"> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> </div> <div class="collapse navbar-collapse" id="myNavbar"> <ul class="nav navbar-nav"> <li><a href="foo.php">Foo</a></li> <li><a href="doo.php">Doo</a></li> </ul> </div> </div> </nav>'; };
Now go back to your header.php and make it look like this.
<?php include_once('functions.php'); echo headtag(); ?> <body> <?php menu(); ?> <div class="main" id="top"> <div class="header"> <img src="logo200.png" id="logo" /> </div>
When you preview the site you will see the new bootstrap menu.
Next Lesson PHP Queries
The next lesson we are going to dive a little further into PHP and queries. I hope you are enjoying these lessons. Please feel free to comment below and like, share and subscribe for more lessons.
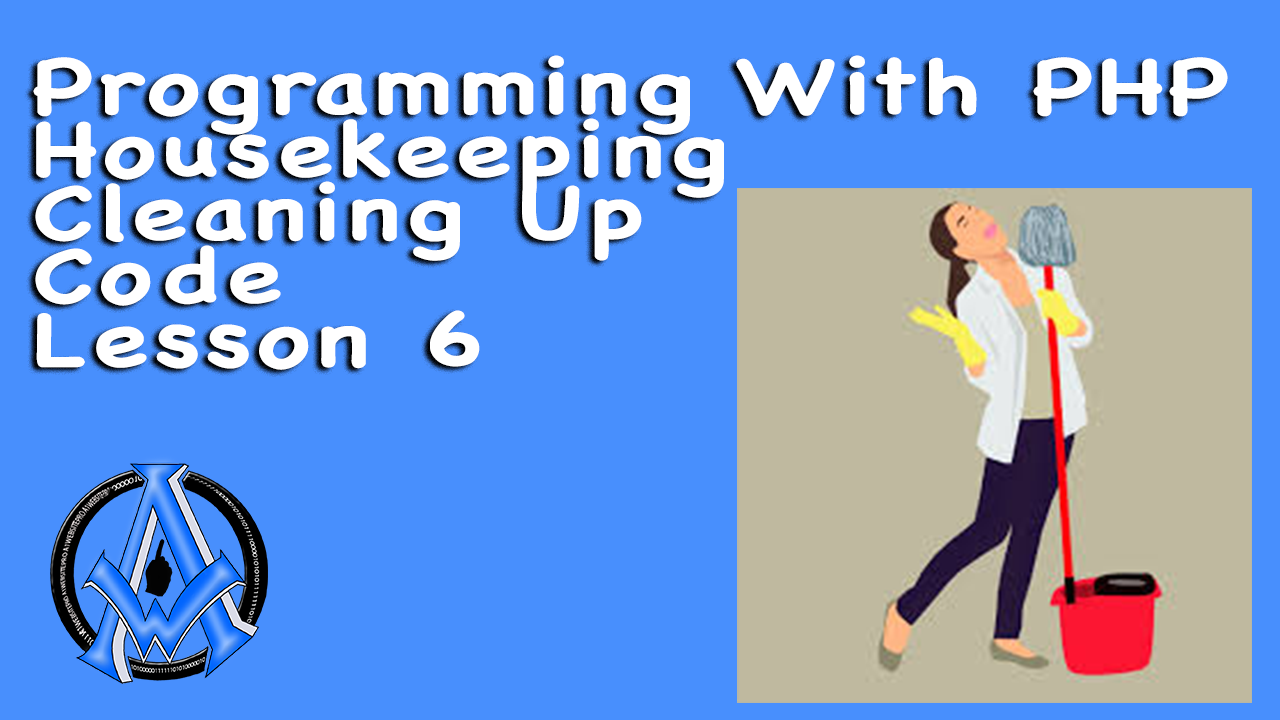
A1WEBSITEPRO Social Media Pages
Here are my social media pages, lets hook up!