Creating The Database
For our example in the video we create a database. We are going to call it “summernote”. You can following along in the video or just enter this code into your sql in phpMyAdmin.
CREATE TABLE `entries` ( `id` int(11) NOT NULL, `content` text NOT NULL, `timestamp` timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp() ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4; ALTER TABLE `entries` ADD PRIMARY KEY (`id`); ALTER TABLE `entries` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT; COMMIT;
Create Index File
Now create a file and call it index.php and paste the following code into it.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Summernote</title> <link href="https://stackpath.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css" rel="stylesheet"> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> <link href="https://cdn.jsdelivr.net/npm/summernote@0.8.18/dist/summernote.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/summernote@0.8.18/dist/summernote.min.js"></script> </head> <body> <h1>Summernote</h1> <form method="post"> <textarea id="editor" name="editordata"></textarea> <button id="save-btn">Save</button> </form> <div id="display"></div>; <script> $(document).ready(function(){ $('#editor').summernote({ height: 300 }); $("#save-btn").click(function(){ var editor = $("#editor").val(); var dataString = 'editor1='+ editor; if(editor=='') { $("#display").html("Please Enter Some Content"); } else { $.ajax({ type: "POST", url: "save.php", data: dataString, cache: false, success: function(result){ $("#display").html(result); } }); } return false; }); }); </script> </body> </html>
Create Save File
Now create a save.php file and copy and paste the following code into it.
<?php $con = mysqli_connect("localhost","root","","summernote"); $content=mysqli_real_escape_string($con, $_POST['editor1']); // Convert special characters to HTML entities $content = htmlspecialchars($content, ENT_QUOTES, 'UTF-8'); $sql = "INSERT INTO entries (content) VALUES ('$content')"; if ($con->query($sql) === TRUE) { echo "New record created successfully"; } else { echo "Error: " . $sql . "<br>" . $con->error; } $con->close(); ?>
Create A Display Page
Now create a display.php page and copy and paste this code into it.
<?php ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); $con = mysqli_connect("localhost","root","","summernote"); // Check connection if ($con->connect_error) { die("Connection failed: " . $con->connect_error); } echo "Connected successfully"; $result = $con->query("SELECT * FROM entries") ; while ($row = $result->fetch_assoc()) { // Decode the HTML entities $decoded_string = html_entity_decode($row['content']); // Output the decoded string echo $decoded_string; //put horizonal line echo '<hr/>'; } $con->close(); ?>
Conclusion
These are the codes that I used in the video. All you should have to do is copy and paste the codes into the files. If you are having any issues comment below. You can also download the summernote files here.

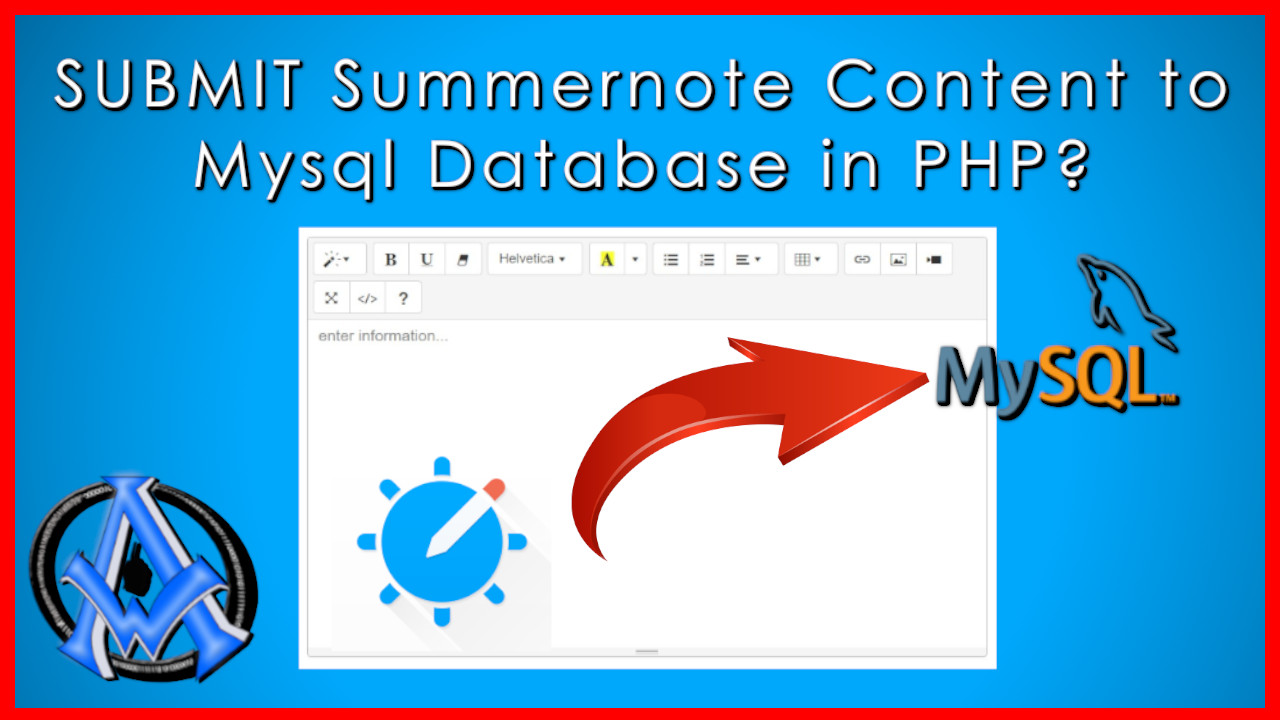

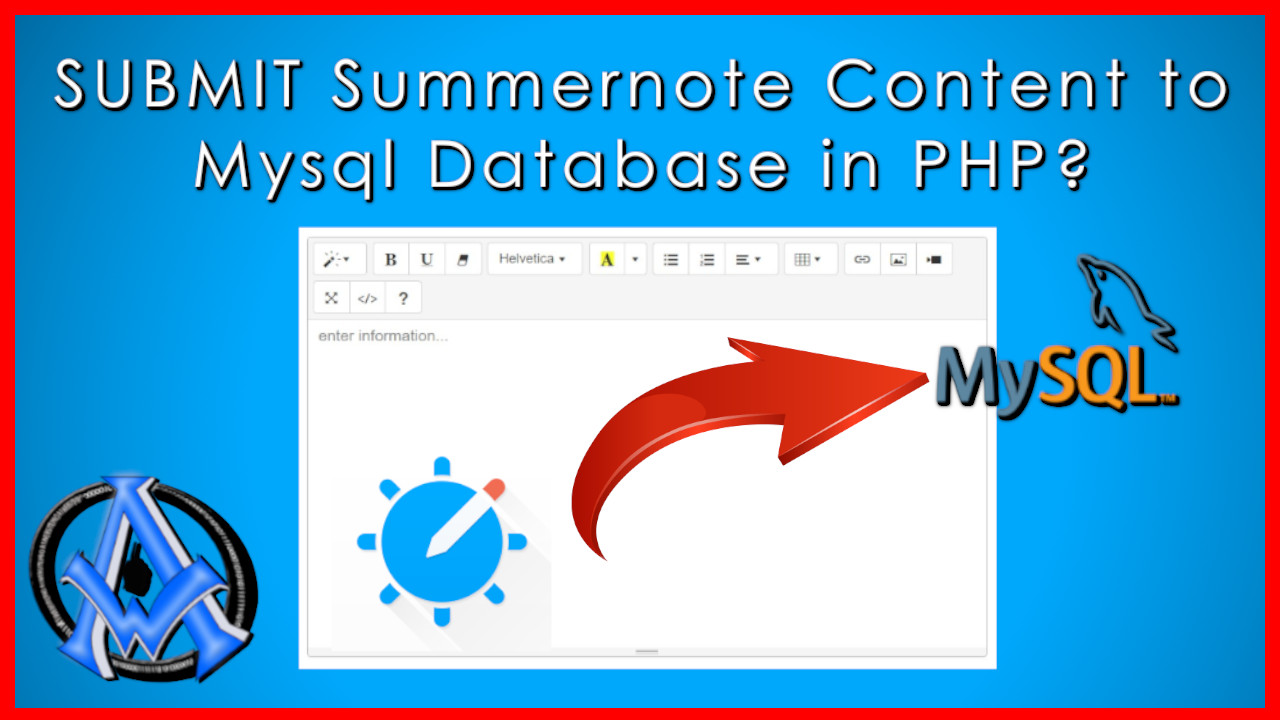
4 Comments
Your videos are really helpful. Thank you.
Would it be possible to create a video showing to read a summernote record from a MySQL database and put it into a summernote so that the text can be edited further, please? Apologies if you have covered this already and my brain cell (singular) missed it.
Thanks again 🙂
HI Andy, if I don’t have it I sure will. Basically it is just updating the database instead of inserting the content. I believe on this on I only showed how to insert the content. https://a1websitepro.com/how-to-submit-summernote-content-to-mysql-database-in-php/ However, I ahve been so busy lately but Ill try to make some time for this.
Thanks for getting back to me. I worked it out and will happily share the code with you. The trick was to make sure that there is no accidental (or intentional) code injection. There is htmlspecialcharaters, but that leaves out some things I would rather have hidden. I wrote my own short routine in PHP to do this (it’s only a .replace() for each character). The Summernote can then be saved in a text field in the ordinary way.
Thank you for sharing. 🙂 Glad you got it.