Download all scripts here for this tutorial.
Create a file and call it index.php. Insert the following code into it and save it. This will be the main file where the process starts.
<!DOCTYPE html> <html lang="en"> <head> <title>Lost Password Script AJAX jQuery Bootstrap PHP and MySql</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <div class="form-group"> <label for="email">Email:</label> <input id="email" class="form-control" type="email" placeholder="Your Email"> </div> <button type="submit" id="submit" class="btn btn-default">Reset Password</button> <div id="display"></div> <script> $(document).ready(function(){ $("#submit").click(function(){ var em = $("#email").val(); var dataString = 'em1='+ em; $.ajax({ type: "POST", url: "processor.php", data: dataString, cache: false, success: function(result){ $("#display").html(result); } }); return false; }); }); </script> </div> </body> </html>
Now create a file and call it processor.php and enter the following code into it.
<?php include_once('config.php'); $email=mysqli_real_escape_string($con, $_POST['em1']); $emailclean = filter_var($email, FILTER_SANITIZE_EMAIL, FILTER_FLAG_STRIP_HIGH); //query the database $result = $con->query("SELECT * FROM users WHERE email='$emailclean' LIMIT 1") ; $total=$result->num_rows; if($total<1){ echo '<div class="alert alert-warning">Are you sure you entered the correct email?</div>'; die(); } while ($row = $result->fetch_assoc()) { $emaill=$row['email']; $password=$row['password']; } //email the form to the person who lost their password $subject='PASSWORD RESET LINK'; $headers = "MIME-Version: 1.0" . "\r\n"; $headers .= "Content-type:text/html;charset=UTF-8" . "\r\n"; $headers .= 'From: <noreply@a1websitepro.com>' . "\r\n"; $message='<table width="100%" border="1"> <tr><td colspan="2" align="center">RESET YOUR PASSWORD BY CLICKING LINK BELOW</td></tr> <tr><td colspan="2" align="center">If you did not make this request then ignore this email.</td></tr><tr><td colspan="2" align="center"><a href="http://localhost/ajax/lostpassword.php?p='.$password.'">Reset Password</a></td></tr> <tr><td colspan="2" align="center"><img src="https://a1websitepro.com/wp-content/uploads/2014/09/logo200.png" width="200px"/></td></tr> </table>'; //mail($emaill,$subject,$message,$headers); //send message back to AJAX echo '<div class="alert alert-success">An email has been sent to '.$email.' with a password reset link.</div>'; $con->close(); ?>
Lost Password Script AJAX jQuery Bootstrap PHP and MySql was last modified: November 14th, 2023 by
Summary
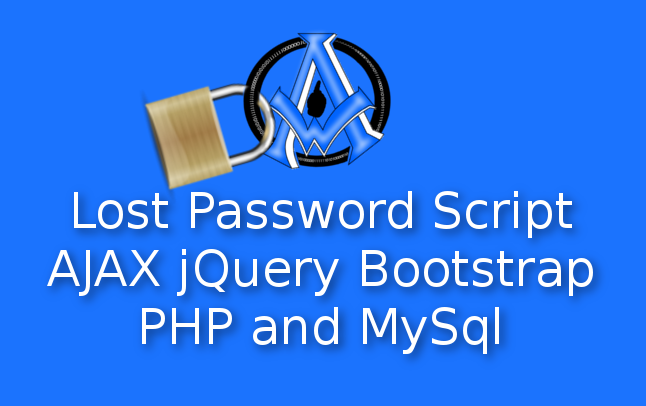
Article Name
Lost Password Script AJAX jQuery Bootstrap PHP and MySql
Description
This is a lost password script tutorial. It is written in AJAX, jQuery, Boostrap, PHP and MySql. Frequently people lose their passwords. Password reset scripts are almost mandatory with people expecting software to do everything for them. Writing scripts that lets people reset their passwords do post some risks.
Author
Maximus McCullough
Publisher
A1WEBSITEPRO LLC
Logo

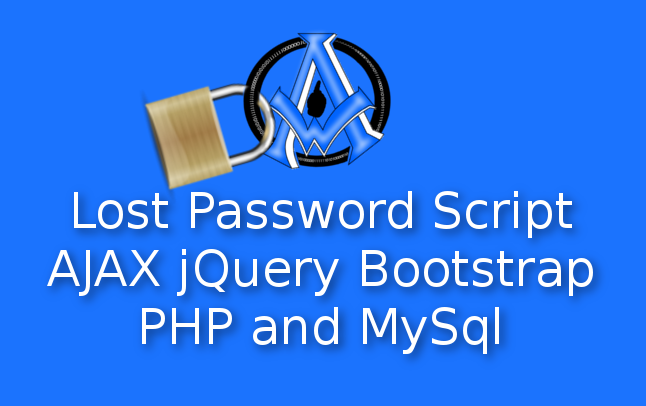
A1WEBSITEPRO Social Media Pages
Here are my social media pages, lets hook up!