Create a database and a table at the same time with php in mysql. All you will need is the connection information to your database. This is what you will need.
Preliminary Information Needed
- Connection to the host. For most of you this will be localhost.
- Mysql username.
- Mysql password.
Applies To
- PHP5 +
- Mysql
You can use phpmyadmin for visual confirmation. However the use of phpmyadmin is not required for this tutorial.
Scripts for PHP lesson.
Create a file and call it createdatabase.php or download it here. Enter the following code in if you are not downloading it.
[php]<?php
include_once(‘db.php’);
// Create connection
$con= new mysqli($localhost, $dbusername, $dbpass);
// Check connection
if ($con->connect_error) {
die("Connection failed: " . $con->connect_error);
}
// Create database
$sql = "CREATE DATABASE $dbname";
if ($con->query($sql) === TRUE) {
echo "Database created successfully";
} else {
echo "Error creating database: " . $con->error;
}
$con = mysqli_connect("$localhost","$dbusername","$dbpass","$dbname");
// sql to create table
$sql = "CREATE TABLE users(
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(250) NOT NULL,
email VARCHAR(250) NOT NULL,
password VARCHAR(250) NOT NULL,
reg_date TIMESTAMP
)";
if ($con->query($sql) === TRUE) {
echo "Table users created successfully";
} else {
echo "Error creating table: " . $con->error;
}
$con->close();
?>[/php]
Create another file and call it db.php. Enter the following code into it. Be sure to change it to your password.
[php]<?php
$localhost=’localhost’;
$dbusername=’root’;
$dbpass=’yourpassword’;
$dbname=’members’;
?>[/php]
Final Steps for Database and Table creation in PHP
Load the createdatabase.php in your browser. If all of your connection information is correct it will create a table called “members” in you database. In the members table you will now have the following.
- id
- username
- password
- reg_date
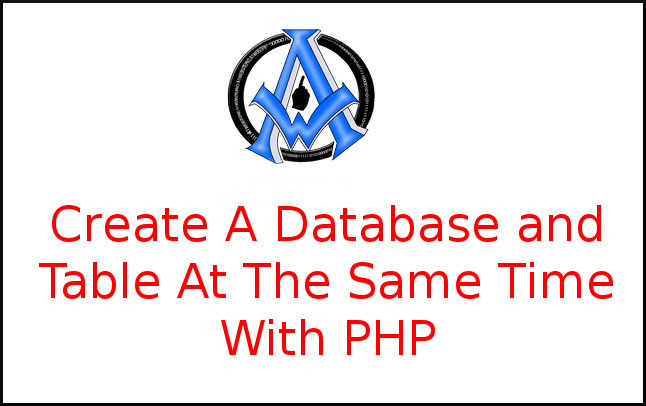

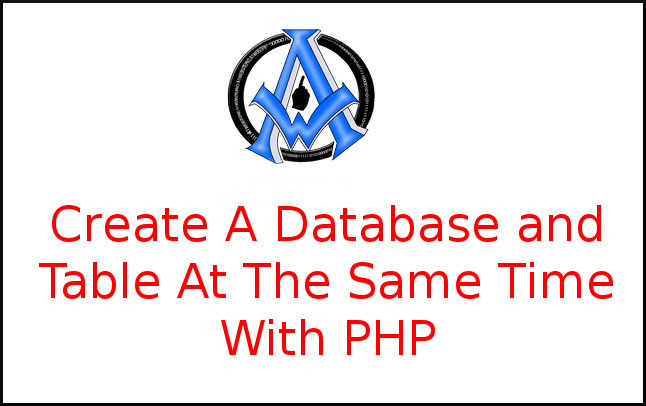
A1WEBSITEPRO Social Media Pages
Here are my social media pages, lets hook up!