If you want to block hackers automatically you have come to the right place. This of course is a PHP and MySql programming tutorial.
Set Up Your Database
The first thing that you have to do is set up your database. If you already have a config file similar to the ones that we show on this website you are ready. If not then make yourself one that connects to your database.
[code]$con = mysqli_connect("localhost","dbusername","dbpass","dbname");
if (mysqli_connect_errno())
{
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}else{
echo "";
}[/code]
Insert New table Into Database
Now you need to create the tables for your database. Create a new file on your server called database.php and put the following code into it.
[code]<?php require(‘config.php’); $sql = "CREATE TABLE block( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, city VARCHAR(250) NOT NULL, region VARCHAR(250) NOT NULL, country VARCHAR(250) NOT NULL, ip VARCHAR(250) NOT NULL, date TIMESTAMP )"; if ($con->query($sql) === TRUE) {
echo "Table block created successfully
";
} else {
echo "Error creating table: " . $con->error;
}
?>[/code]
Create a Block.php Page
Now create a new file and call it block.php on your server. Insert the following code below. Make sure that you replace http://yourwebsite.com with whatever your website is called.
[code]<?php $theurl= $_SERVER["HTTP_REFERER"]; if ($urlParts = parse_url($theurl)) $baseUrl = $urlParts["scheme"] . "://" . $urlParts["host"]; if($baseUrl==’http://yourwebsite.com’){ echo ”; }else{ echo ‘Hi There, nice job on the Hacking! However as you can see I have your information. I am either ready to report your actions to the authorities or you can email me and have a little business discussion. I have some work for you. If I do not hear from you within 24 hours then I will report you to the closest authorities. You are of course blocked now from the site. I do know that there are other means of accessing but I would not try that if I were you. ‘; if (!empty($_SERVER[‘HTTP_CLIENT_IP’])) { $ip = $_SERVER[‘HTTP_CLIENT_IP’]; } elseif (!empty($_SERVER[‘HTTP_X_FORWARDED_FOR’])) { $ip = $_SERVER[‘HTTP_X_FORWARDED_FOR’]; } else { $ip = $_SERVER[‘REMOTE_ADDR’]; } echo ‘ ‘; $geo = unserialize(file_get_contents("http://www.geoplugin.net/php.gp?ip=$ip")); $city = $geo["geoplugin_city"]; $region = $geo["geoplugin_regionName"]; $country = $geo["geoplugin_countryName"]; $geoplugin_request = $geo["geoplugin_request"]; $geoplugin_status = $geo["geoplugin_status"]; $geoplugin_region = $geo["geoplugin_region"]; $geoplugin_areaCode = $geo["geoplugin_areaCode"]; $geoplugin_dmaCode = $geo["geoplugin_dmaCode"]; $geoplugin_countryCode = $geo["geoplugin_countryCode"]; $geoplugin_countryName = $geo["geoplugin_countryName"]; $geoplugin_continentCode = $geo["geoplugin_continentCode"]; $geoplugin_latitude = $geo["geoplugin_latitude"]; $geoplugin_longitude = $geo["geoplugin_longitude"]; $geoplugin_regionCode = $geo["geoplugin_regionCode"]; $geoplugin_regionName = $geo["geoplugin_regionName"]; $geoplugin_currencyCode = $geo["geoplugin_currencyCode"]; $geoplugin_currencySymbol = $geo["geoplugin_currencySymbol"]; $geoplugin_currencySymbol_UTF8 = $geo["geoplugin_currencySymbol_UTF8"]; $geoplugin_currencyConverter = $geo["geoplugin_currencyConverter"]; include(‘config.php’); mysqli_query($con,"INSERT INTO block(`city`, `region`, `country`,`ip`)VALUES(‘$city’,’$region’,’$country’,’$geoplugin_request’)"); echo " City: ".$city." "; echo "Region: ".$region." "; echo "Country: ".$country." "; echo "IP: ".$geoplugin_request." "; echo "Status: ".$geoplugin_status." "; echo "Region: ".$geoplugin_region." "; echo "Area Code: ".$geoplugin_areaCode." "; echo "DMA Code: ".$geoplugin_dmaCode." "; echo "Country Code: ".$geoplugin_countryCode." "; echo "Continent Code: ".$geoplugin_continentCode." "; echo "Latitude: ".$geoplugin_latitude." "; echo "Longitude: ".$geoplugin_longitude." "; echo "Region Code: ".$geoplugin_regionCode." "; echo "Region Name: ".$geoplugin_regionName." "; echo "Currency Code: ".$geoplugin_currencyCode." "; echo "Currency Symbol: ".$geoplugin_currencySymbol." "; echo "Currency Symbol UTF8: ".$geoplugin_currencySymbol_UTF8." "; echo "Currency Converter: ".$geoplugin_currencyConverter." "; die(); }; ?>[/code]
What does this Script Do?
The first thing that this script does is it looks to make sure that this particular page was accessed from somewhere on your website. Many hackers like to go to where you have your insert scripts into your database. If they did not come from your website this script then takes action. If they did come from your website then the script will act accordingly they way it does for your customers.
The second thing this script does is give your little hacker buddy a message. You can change the text around to say whatever you want.
The third thing that it does is gets your hacker buddies information. We do this with the geo plugin. You can get all kids of information about the hackers location and act accordingly. Later on in this lesson we are going to auto block them from accessing the website. Basically all we need it their ip to do this.
The fourth thing this script does is stores all the information from the hacker into your database so we can call it and block him later when he tries to come back.
Where to include the block.php script.
It is important that you understand this so read! You only want to put this script in confirmation or submission pages. The pages that are responsible for submitting things to your database. Watch the video for detailed instructions. You do NOT want to put this in your header.php.
This is how you include the block.php page.
[code]<?php include(‘block.php’);?>[/code]
Add This to Your header.php page
So now we have to block the nasty hackers from your entire website. You need to add the following code to your header.php.
[code]<?php require(‘config.php’); ?>
<?php if (!empty($_SERVER[‘HTTP_CLIENT_IP’])) { $ip = $_SERVER[‘HTTP_CLIENT_IP’]; } elseif (!empty($_SERVER[‘HTTP_X_FORWARDED_FOR’])) { $ip = $_SERVER[‘HTTP_X_FORWARDED_FOR’]; } else { $ip = $_SERVER[‘REMOTE_ADDR’]; } $resultb = $con->query("SELECT * FROM block") ;
while ($row = $resultb->fetch_assoc()) {
if($ip==$row[‘ip’]){
header("location: http://www.google.com/");
exit();
}else{
echo ”;
}
};
?>[/code]
Congratulations, now you can block them nasty hackers. The other thing that this script will do is save you on your SMTP relays. Hackers that get a hold of your submission scripts can work havoc on your emails. If you are on a shared server they can burn up quick.
I hope you enjoyed this tutorial. If you have any questions feel free to comment below.
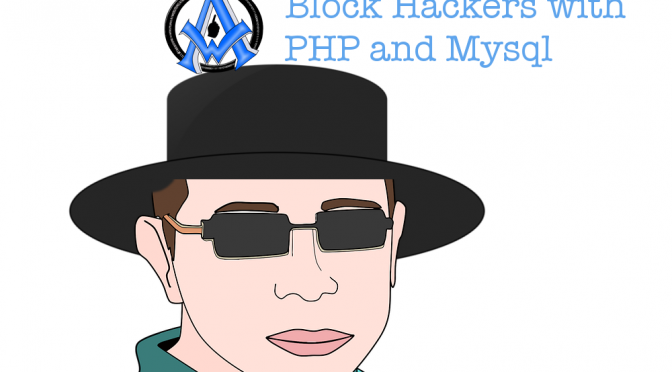
3 Comments
Please clarify for me what header.php is?
The header.php is the top part of your website. This files is usually responsible for meta tags, scripts, style sheets and many other things.
Hey thanks, thought so LOL. I am trying to implement this. However when I attempt to visit the website it believes I am a hacker?? I have updated my url to the correct domain so am unsure of when its going wrong.